Debugging tools
Playwright scripts work with existing debugging tools, like Node.js debuggers and browser developer tools. Playwright also introduces new debugging features for browser automation.
- Playwright Inspector
- Playwright Trace Viewer
- Run in headed mode
- Browser Developer Tools
- Run in Debug Mode
- Selectors in Developer Tools Console
- Visual Studio Code debugger (Node.js)
- Verbose API logs
#
Playwright InspectorPlaywright Inspector is a GUI tool that helps authoring and debugging Playwright scripts. That's our default recommended tool for scripts troubleshooting.
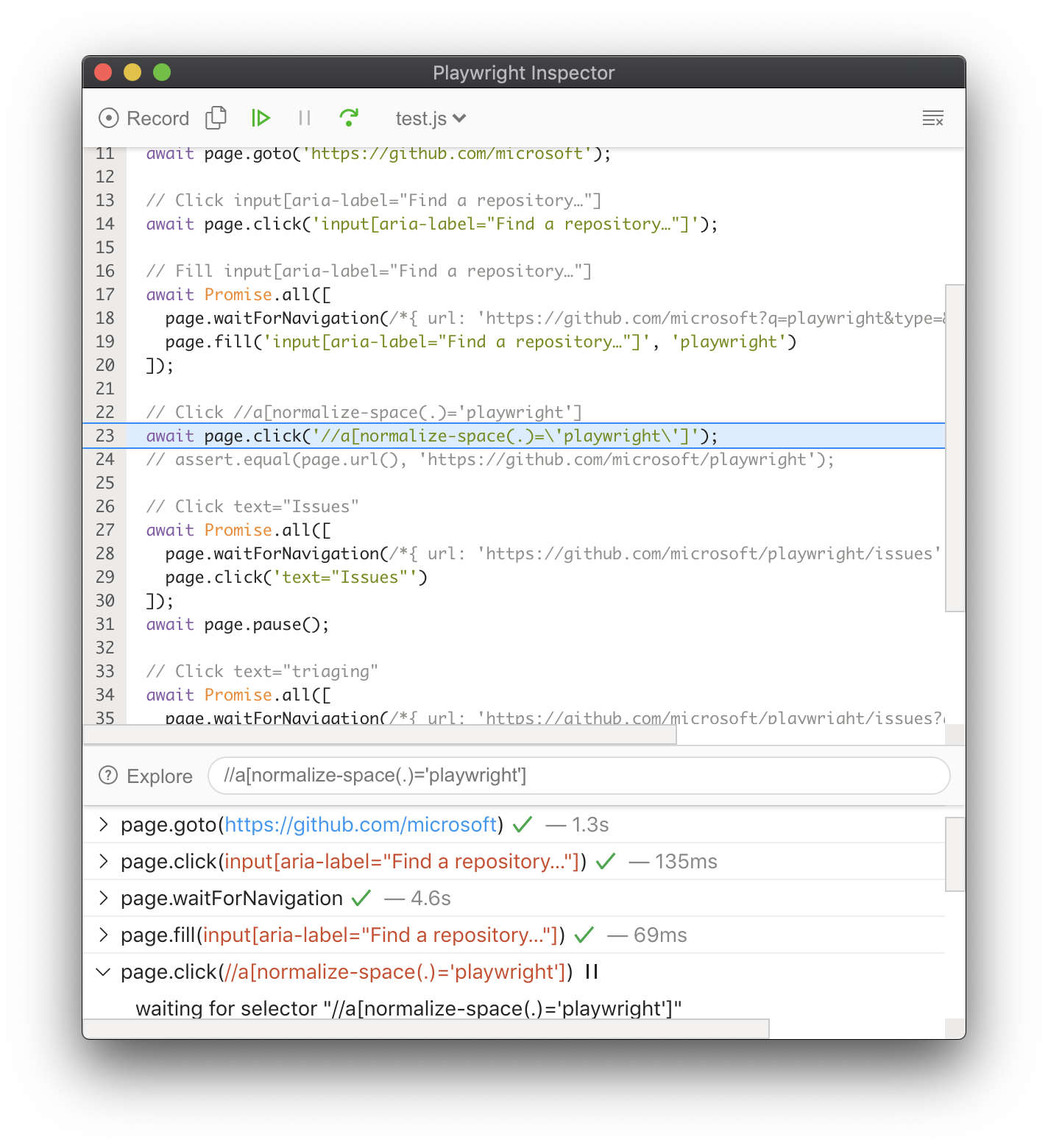
#
Playwright Trace ViewerPlaywright Trace Viewer is a GUI tool that helps troubleshooting test runs in a post-mortem manner.
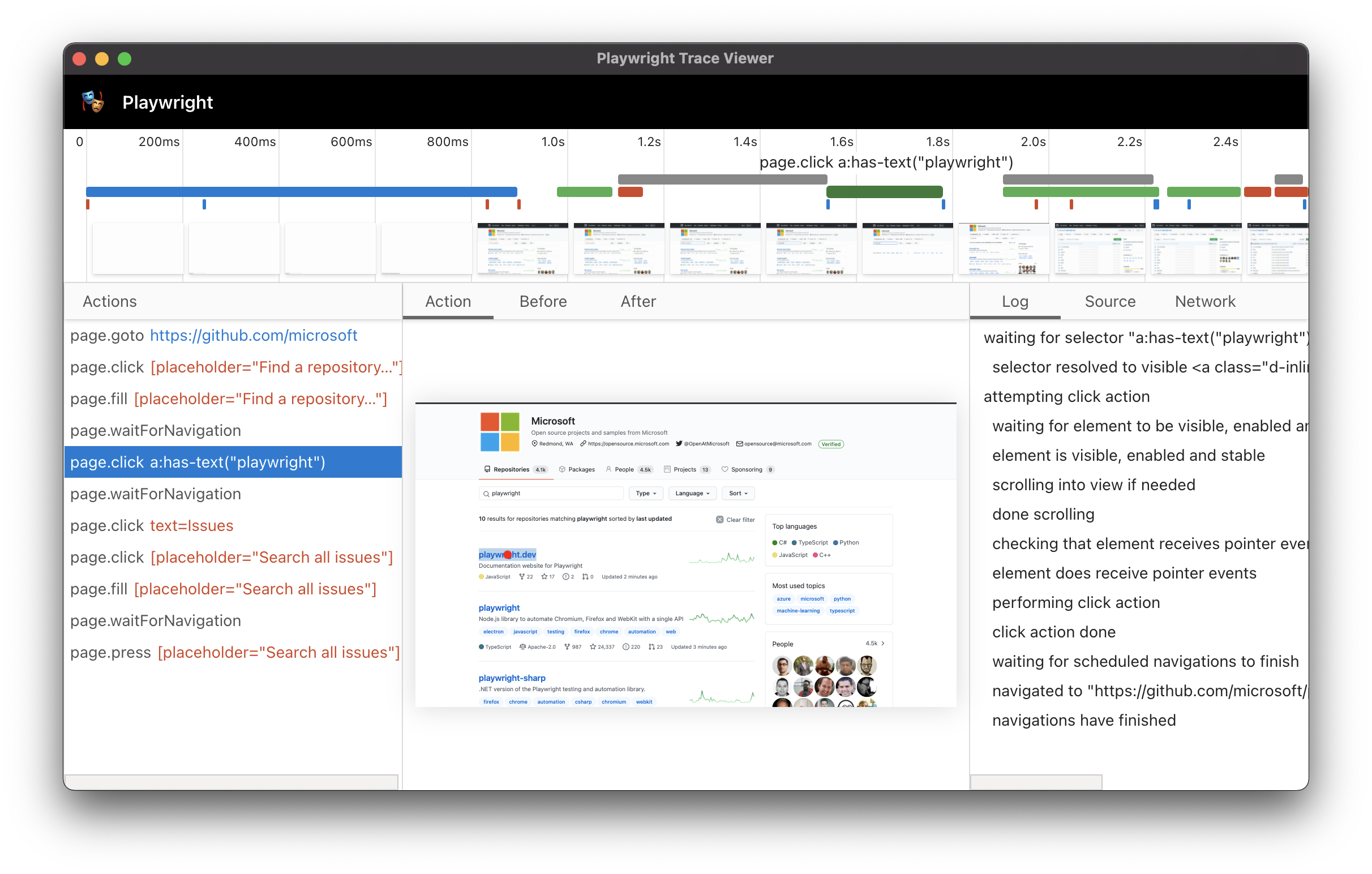
#
Run in headed modePlaywright runs browsers in headless mode by default. To change this behavior, use headless: false
as a launch option. You can also use the slowMo
option to slow down execution and follow along while debugging.
await chromium.launch({ headless: false, slowMo: 100 }); // or firefox, webkit
#
Browser Developer ToolsYou can use browser developer tools in Chromium, Firefox and WebKit while running a Playwright script in headed mode. Developer tools help to:
- Inspect the DOM tree and find element selectors
- See console logs during execution (or learn how to read logs via API)
- Check network activity and other developer tools features
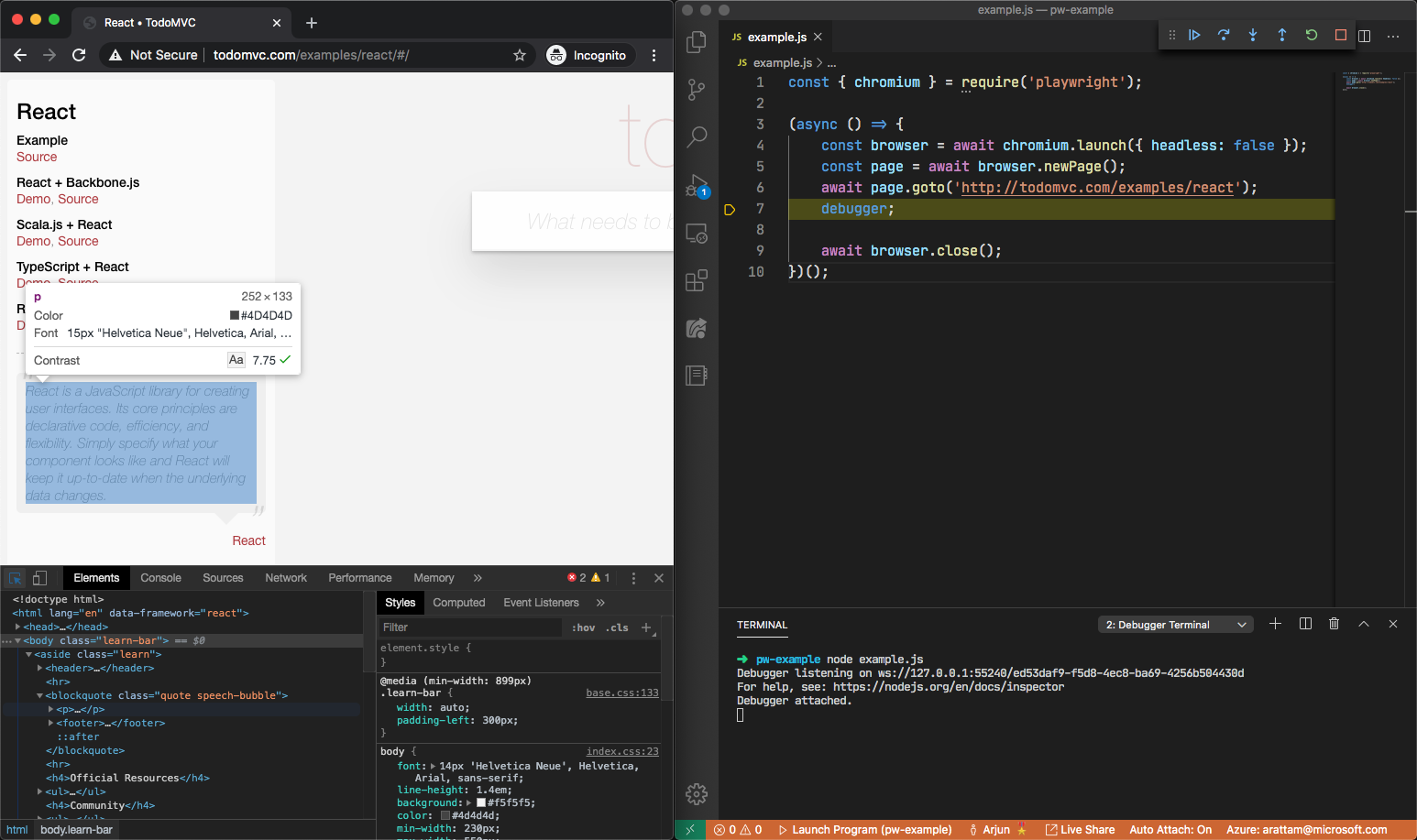
Using a page.pause() method is an easy way to pause the Playwright script execution and inspect the page in Developer tools. It will also open Playwright Inspector to help with debugging.
For Chromium: you can also open developer tools through a launch option.
await chromium.launch({ devtools: true });
note
For WebKit: launching WebKit Inspector during the execution will prevent the Playwright script from executing any further.
#
Run in Debug ModeSet the PWDEBUG
environment variable to run your scripts in debug mode. Using PWDEBUG=1
will open Playwright Inspector.
Using PWDEBUG=console
will configure the browser for debugging in Developer tools console:
- Runs headed: Browsers always launch in headed mode
- Disables timeout: Sets default timeout to 0 (= no timeout)
- Console helper: Configures a
playwright
object in the browser to generate and highlight Playwright selectors. This can be used to verify text or composite selectors.
# Linux/macOSPWDEBUG=console npm run test
# Windows with cmd.exeset PWDEBUG=consolenpm run test
# Windows with PowerShell$env:PWDEBUG="console"npm run test
#
Selectors in Developer Tools ConsoleWhen running in Debug Mode with PWDEBUG=console
, a playwright
object is available in Developer tools console.
- Run with
PWDEBUG=console
- Setup a breakpoint to pause the execution
- Open the console panel in browser developer tools
- Use the
playwright
APIplaywright.$(selector)
: Highlight the first occurrence of the selector. This reflects howpage.$
would see the page.playwright.$$(selector)
: Highlight all occurrences of the selector. This reflects howpage.$$
would see the page.playwright.inspect(selector)
: Inspect the selector in the Elements panel.playwright.clear()
: Clear existing highlights.playwright.selector(element)
: Generate a selector that points to the element.
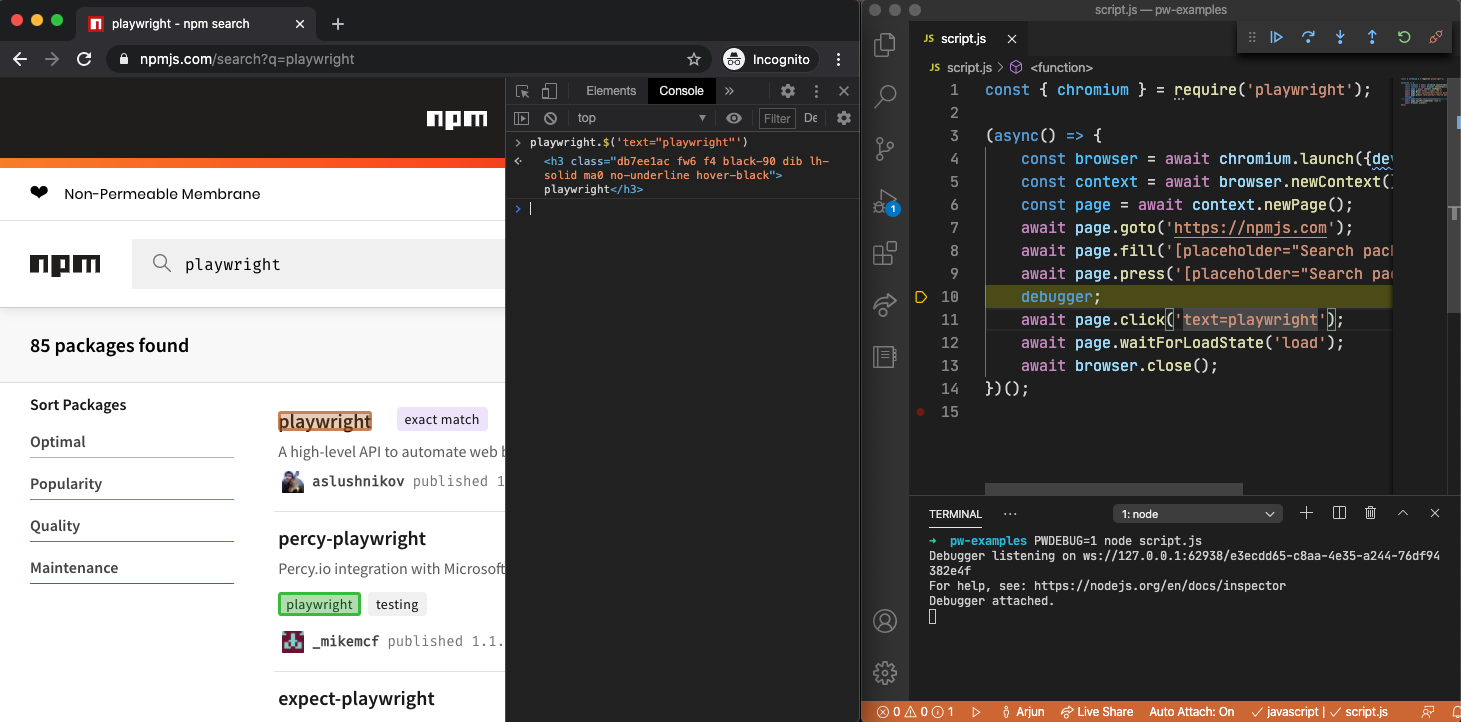
#
Visual Studio Code debugger (Node.js)The VS Code debugger can be used to pause and resume execution of Playwright scripts with breakpoints. The debugger can be configured in two ways.
#
Use launch configSetup launch.json
configuration for your Node.js project. Once configured launch the scripts with F5 and use breakpoints.
#
Use the JavaScript Debug Terminal- Open JavaScript Debug Terminal
- Set a breakpoint in VS Code
- Use the
debugger
keyword or set a breakpoint in the VS Code UI
- Use the
- Run your Node.js script from the terminal
#
Verbose API logsPlaywright supports verbose logging with the DEBUG
environment variable.
# Linux/macOSDEBUG=pw:api npm run test
# Windows with cmd.exeset DEBUG=pw:apinpm run test
# Windows with PowerShell$env:DEBUG="pw:api"npm run test