Command line tools
Playwright comes with the command line tools.
- Usage
- Install browsers
- Generate code
- Open pages
- Inspect selectors
- Take screenshot
- Generate PDF
- Install system dependencies
#
Usagenpx playwright --help
# Running from `package.json` script{ "scripts": { "help": "playwright --help" }}
#
Install browsersPlaywright can install supported browsers.
# Running without arguments will install default browsersnpx playwright install
You can also install specific browsers by providing an argument:
# Install WebKitnpx playwright install webkit
See all supported browsers:
npx playwright install --help
#
Generate codenpx playwright codegen wikipedia.org
Run codegen
and perform actions in the browser. Playwright CLI will generate JavaScript code for the user interactions. codegen
will attempt to generate resilient text-based selectors.
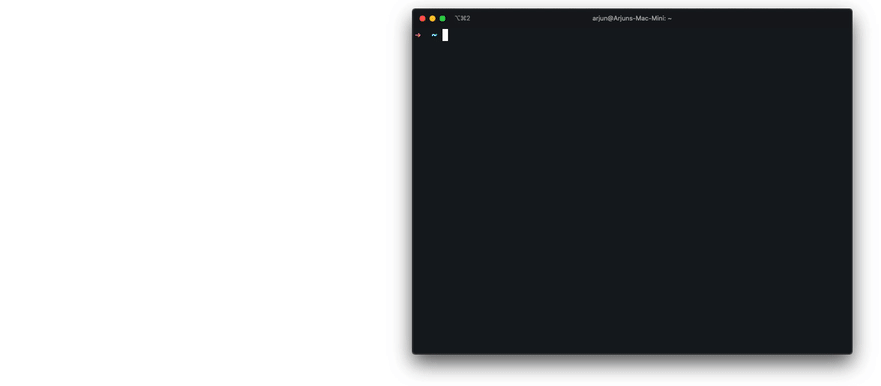
#
Preserve authenticated stateRun codegen
with --save-storage
to save cookies and localStorage at the end. This is useful to separately record authentication step and reuse it later.
npx playwright codegen --save-storage=auth.json# Perform authentication and exit.# auth.json will contain the storage state.
Run with --load-storage
to consume previously loaded storage. This way, all cookies and localStorage will be restored, bringing most web apps to the authenticated state.
npx playwright open --load-storage=auth.json my.web.appnpx playwright codegen --load-storage=auth.json my.web.app# Perform actions in authenticated state.
#
Codegen with custom setupIf you would like to use codegen in some non-standard setup (for example, use browserContext.route(url, handler[, options])), it is possible to call page.pause() that will open a separate window with codegen controls.
const { chromium } = require('playwright');
(async () => { // Make sure to run headed. const browser = await chromium.launch({ headless: false });
// Setup context however you like. const context = await browser.newContext({ /* pass any options */ }); await context.route('**/*', route => route.continue());
// Pause the page, and start recording manually. const page = await context.newPage(); await page.pause();})();
#
Open pagesWith open
, you can use Playwright bundled browsers to browse web pages. Playwright provides cross-platform WebKit builds that can be used to reproduce Safari rendering across Windows, Linux and macOS.
# Open page in Chromiumnpx playwright open example.com
# Open page in WebKitnpx playwright wk example.com
#
Emulate devicesopen
can emulate mobile and tablet devices from the playwright.devices
list.
# Emulate iPhone 11.npx playwright open --device="iPhone 11" wikipedia.org
#
Emulate color scheme and viewport size# Emulate screen size and color scheme.npx playwright open --viewport-size=800,600 --color-scheme=dark twitter.com
#
Emulate geolocation, language and timezone# Emulate timezone, language & location# Once page opens, click the "my location" button to see geolocation in actionnpx playwright open --timezone="Europe/Rome" --geolocation="41.890221,12.492348" --lang="it-IT" maps.google.com
#
Inspect selectorsDuring open
or codegen
, you can use following API inside the developer tools console of any browser.
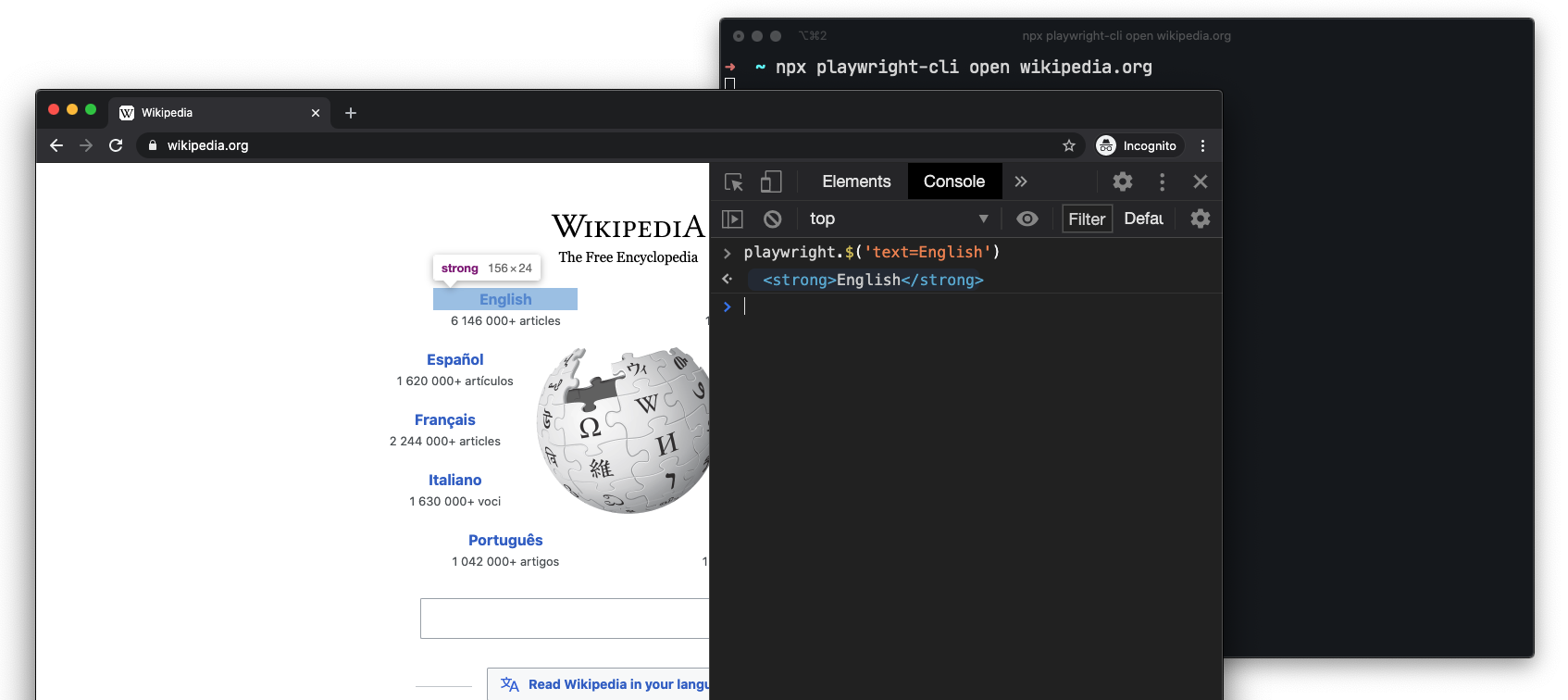
#
playwright.$(selector)Query Playwright selector, using the actual Playwright query engine, for example:
> playwright.$('.auth-form >> text=Log in');
<button>Log in</button>
#
playwright.$$(selector)Same as playwright.$
, but returns all matching elements.
> playwright.$$('li >> text=John')
> [<li>, <li>, <li>, <li>]
#
playwright.inspect(selector)Reveal element in the Elements panel (if DevTools of the respective browser supports it).
> playwright.inspect('text=Log in')
#
playwright.selector(element)Generates selector for the given element.
> playwright.selector($0)
"div[id="glow-ingress-block"] >> text=/.*Hello.*/"
#
Take screenshot# See command helpnpx playwright screenshot --help
# Wait 3 seconds before capturing a screenshot after page loads ('load' event fires)npx playwright screenshot \ --device="iPhone 11" \ --color-scheme=dark \ --wait-for-timeout=3000 \ twitter.com twitter-iphone.png
# Capture a full page screenshotnpx playwright screenshot --full-page en.wikipedia.org wiki-full.png
#
Generate PDFPDF generation only works in Headless Chromium.
# See command helpnpx playwright pdf https://en.wikipedia.org/wiki/PDF wiki.pdf
#
Install system dependenciesUbuntu 18.04 and Ubuntu 20.04 system dependencies can get installed automatically. This is useful for CI environments.
# See command helpnpx playwright install-deps
You can also install the dependencies for a single browser only by passing it as an argument:
npx playwright install-deps chromium